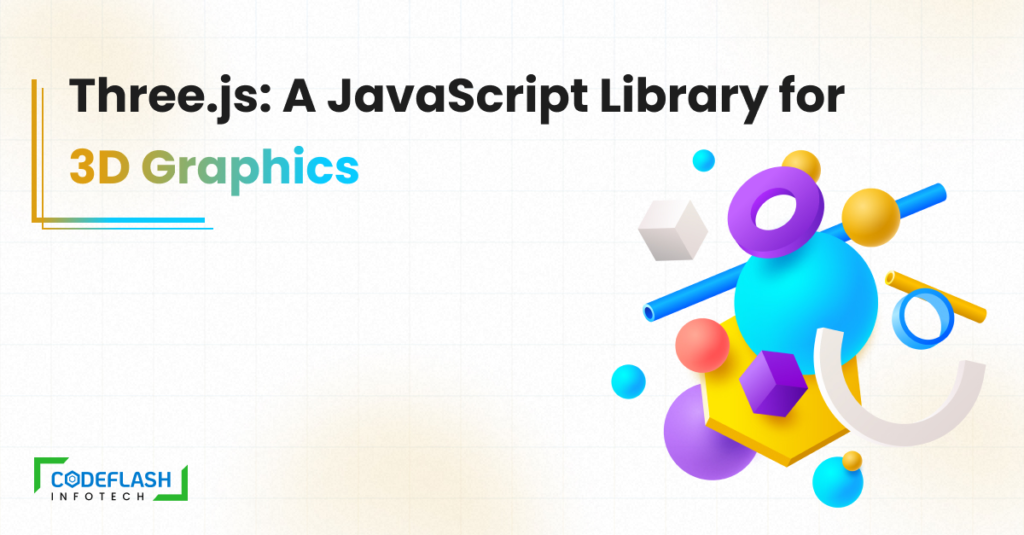
Three.js: A JavaScript Library for 3D Graphics
Written by: Dhaval Sejani | 22 DECEMBER
Three.js: A JavaScript Library for 3D Graphics
Suppose you want to create and display interactive 3D graphics in web browsers. In that case, you might want to check out Three.js, an open-source JavaScript library that simplifies working with WebGL, a technology that enables hardware-accelerated rendering of 3D graphics in browsers.
In this blog, we will give you an overview of what Three js A JavaScript is, what it can do, and how to use it. We will also show you a simple demo of creating a spinning cube with Three.js and discuss some advantages and disadvantages of using this library.
What is Three js A JavaScript?
Three.js library provides tools and features to make 3D graphics development more accessible and user-friendly. With Three.js, you can easily create and manipulate 3D scenes, objects, lights, cameras, textures, materials, and animations. You can also load and display 3D models in other 3D modelling software.
Three.js is based on WebGL, a web standard that allows browsers to access a computer’s graphics processing unit (GPU) and render 3D graphics at high speed. However, WebGL is a low-level API requiring a lot of code and technical knowledge. Three.js abstracts away the complexity of WebGL and provides a higher-level API that is easier to work with.
Some examples of what you can do with Three.js are:
- Create a simple 3D scene with a cube and a sphere.
- Load and display a 3D model of a car.
- Create an animation of a ball bouncing.
- Create a simulation of a planet orbiting a star.
- Create a visualization of a data set.
How to use Three.js in JavaScript?
To use Three.js, you must include the library in your HTML file from a content delivery network (CDN) or download it from the official website. Then, you can write your JavaScript code to create and render your 3D scene.
The basic steps to create a 3D scene with Three.js are:
- Create a scene object that contains all the elements of your 3D world.
- Create a camera object that defines how you view the scene.
- Create a renderer object that draws the scene onto a canvas element in your HTML file.
- Create one or more mesh objects that combine the geometry (the shape) and a material (the appearance) of your 3D objects.
- Add the mesh objects to the scene object.
- Position the camera and the mesh objects as desired.
- Create an animation loop that updates and renders the scene continuously.
A simple Three.js demo
To demonstrate how to use Three.js, we will show you how to create a spinning cube on the screen. Here are the steps:
- Create an HTML file (e.g., index.html) and open it in a text editor or an IDE.
- Include the Three.js library from a CDN by adding the following code within the <head> section of your HTML file:
<script src=”https://cdnjs.cloudflare.com/ajax/libs/three.js/r128/three.min.js”></script> - Add a <script> tag within the <body> section of your HTML file to write the JavaScript code:
// Create a scene
const scene = new THREE.Scene();// Create a camera
const camera = new THREE.PerspectiveCamera(
75,
window.innerWidth / window.innerHeight,
0.1,
1000
);camera.position.z = 5;
// Create a renderer
const renderer = new THREE.WebGLRenderer();
renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(renderer.complement);
// Create a cube
const geometry = new THREE.BoxGeometry();
const material = new THREE.MeshBasicMaterial({ color: 0x00ff00 });
const cube = new THREE.Mesh(geometry, material);
scene.add(cube);// Animation loop
function animate() {
requestAnimationFrame(animate);// Rotate the cube
cube.rotation.x += 0.01;
cube.rotation.y += 0.01;// Render the scene
renderer.render(scene, camera);
}// Start the animation loop
animate(); - Save the HTML file and open it in a web browser. You should see a spinning green cube on the screen.
Advantages and disadvantages of Three.js
Three.js is a powerful library that can create various 3D graphics, including games, simulations, and visualizations. It is a popular choice among web developers and 3D artists, and many large companies, such as Google, Facebook, and Autodesk, use it.
Some of the advantages of using Three.js are:
- It hides the various details of WebGL and makes it easier to work with.
- It supports most web browsers and requires no client or plugin to add 3D graphics.
- It provides access to the power of a computer’s graphics and enables high-performance rendering of 3D graphics.
- It offers comprehensive tools and features to create and manipulate 3D scenes, objects, lights, cameras, textures, materials, and animations.
Some of the disadvantages of using Three.js are:
- It depends on OpenGL 2.0 drivers, which may not be available on some devices or browsers.
- It is still at an early stage of development and may have some bugs or compatibility issues.
- It may be slow or inefficient for some complex or large-scale 3D applications.
- It requires some familiarity with 3D graphics concepts and JavaScript programming.
Conclusion
Three.js is a JavaScript library that allows you to create and display interactive 3D graphics in web browsers. It simplifies working with WebGL, a technology that enables hardware-accelerated rendering of 3D graphics in browsers. With Three.js, you can easily create and manipulate 3D scenes, objects, lights, cameras, textures, materials, and animations. You can also load and display 3D models in other 3D modeling software.
Three.js is a powerful library that can create various 3D graphics, including games, simulations, and visualizations. However, it has limitations and challenges you should know before using it.
To learn more about Three.js, you can visit the official website, where you can find documentation, examples, tutorials, and forums. You can also check out online courses or books that teach you how to use Three.js for different projects.
We hope this blog has given you a basic introduction to Three.js and how to use it. If you have any questions or feedback, feel free to comment below. Happy coding! Discover more blog posts by exploring our website and delving into Our Knowledge Bank.